MVC Crud Operation with Interfaces and Repository Pattern with ADO.Net
Step 1: Open the SQL Server and create a database table and related stored procedure.
/* Create Table Customer */
CREATE TABLE [dbo].[Customers](
[CustomerId] [int] IDENTITY(1,1) NOT NULL,
[FirstName] [nvarchar](250) NULL,
[LastName] [nvarchar](250) NULL,
[Phone] [nvarchar](20) NULL,
[Email] [nvarchar](250) NULL,
[Street] [nvarchar](250) NULL,
[City] [nvarchar](250) NULL,
[State] [nvarchar](250) NULL,
[Zipcode] [nvarchar](10) NULL,
CONSTRAINT [PK_Customers] PRIMARY KEY CLUSTERED
(
[CustomerId] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
/* TO GET Customer BY ID */
CREATE procedure [dbo].[GetCustomersById]
@CustomerId int
as
begin
Select * from Customers
where CustomerId=@CustomerId
end
/* TO Create New Customer */
create procedure [dbo].[InsertCustomers]
@FirstName nvarchar(250),
@LastName nvarchar(250),
@Phone nvarchar(20),
@Email nvarchar(250),
@Street nvarchar(250),
@City nvarchar(250),
@State nvarchar(250),
@Zipcode nvarchar(10)
as
begin
insert into Customers (FirstName,LastName,Phone,Email,Street,City,State,Zipcode) values (@FirstName,@LastName,@Phone,@Email,@Street,@City,@State,@Zipcode)
end
/* TO UPDATE Customer */
create procedure [dbo].[UpdateCustomers]
@CustomerId int ,
@FirstName nvarchar(250),
@LastName nvarchar(250),
@Phone nvarchar(20),
@Email nvarchar(250),
@Street nvarchar(250),
@City nvarchar(250),
@State nvarchar(250),
@Zipcode nvarchar(10)
as
begin
update Customers set FirstName=@FirstName,LastName=@LastName,Phone=@Phone,Email=@Email,Street=@Street,City=@City,State=@State,Zipcode=@Zipcode where CustomerId=@CustomerId
end
/* T0 Delete Customer */
create procedure [dbo].[DeleteCustomers]
@CustomerId int
as
begin
delete Customers where CustomerId=@CustomerId
end
/* To Get All Customer */
CREATE procedure [dbo].[GetCustomers]
as
begin
Select * from Customers
end
Step 2: Create a project
- Start Visual Studio 2017 or 2015.
- Create a new project -> Web -> Visual Studio 2017.
3. Select ASP.NET Web Application(.Net Framework).
4.Provide the Name and Location for the project and click Next.
5. Choose an "Empty" template and check "MVC" under "Add folders & core references" then click Ok.
Step 3: Right-click on the Models folder select "Add" then "Choose a class" window will appear. From that window, select Visual C# and Class then give a class name as "Customer.cs" Then click "Add".
Customer Class
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Web;
namespace MvcWithRepositry.Models
{
public class Customer
{
public int CustomerId { get; set; }
[Required]
[Display(Name = "First Name")]
public string FirstName { get; set; }
[Required]
[Display(Name = "Last Name")]
public string LastName { get; set; }
[Required]
[Display(Name = "Phone")]
public string Phone { get; set; }
[Required]
[Display(Name = "Email")]
public string Email { get; set; }
[Required]
[Display(Name = "Street")]
public string Street { get; set; }
[Required]
[Display(Name = "City")]
public string City { get; set; }
[Required]
[Display(Name = "State")]
public string State { get; set; }
[Required]
[Display(Name = "Zipcode")]
public string Zipcode { get; set; }
}
}
Step 4: Right-click on the project "Add" folder name Repository then Right-click on the Repository folder select "Add" then "Choose a class" window will appear. From that window, select Visual C# and then see on right side Class will display select class and then give a class name as "RespoCustomer.cs" Then click "Add". and after that again right click on Repository folder "Add" an interface ICustomer.cs .
ICustomer interface
using MvcWithRepositry.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MvcWithRepositry.Repository
{
interface ICustomer
{
IList<Customer> GetCustomers();
Customer GetCustomerById(int? id);
void InsertCustomer(Customer customer);
void UpdateCustomer(Customer customer);
void DeleteCustomer(int? id);
}
}
RespoCustomer Class
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Web;
using MvcWithRepositry.Models;
namespace MvcWithRepositry.Repository
{
public class RespoCustomer : ICustomer
{
private readonly string CS = ConfigurationManager.ConnectionStrings["connectionString"].ConnectionString;
public void DeleteCustomer(int? id)
{
using (SqlConnection con = new SqlConnection(CS))
{
var cmd = new SqlCommand("DeleteCustomers", con);
cmd.CommandType = CommandType.StoredProcedure;
con.Open();
cmd.Parameters.AddWithValue("@CustomerId", id);
cmd.ExecuteNonQuery();
}
}
public Customer GetCustomerById(int? id)
{
Customer customers = new Customer();
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("GetCustomersById", con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("CustomerId", id);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
customers.CustomerId = Convert.ToInt32(rdr["CustomerId"]);
customers.FirstName = Convert.ToString(rdr["FirstName"]);
customers.LastName = Convert.ToString(rdr["LastName"]);
customers.Phone = Convert.ToString(rdr["Phone"]);
customers.Email = Convert.ToString(rdr["Email"]);
customers.Street = Convert.ToString(rdr["Street"]);
customers.City = Convert.ToString(rdr["City"]);
customers.State = Convert.ToString(rdr["State"]);
customers.Zipcode = Convert.ToString(rdr["Zipcode"]);
}
return customers;
}
}
public IList<Customer> GetCustomers()
{
List<Customer> customers = new List<Customer>();
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("GetCustomers", con);
cmd.CommandType = CommandType.StoredProcedure;
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
var customer = new Customer()
{
CustomerId = Convert.ToInt32(rdr["CustomerId"]),
FirstName = Convert.ToString(rdr["FirstName"]),
LastName = Convert.ToString(rdr["LastName"]),
Phone = Convert.ToString(rdr["Phone"]),
Email = Convert.ToString(rdr["Email"]),
Street = Convert.ToString(rdr["Street"]),
City = Convert.ToString(rdr["City"]),
State = Convert.ToString(rdr["State"]),
Zipcode = Convert.ToString(rdr["Zipcode"]),
};
customers.Add(customer);
}
return (customers);
}
}
public void InsertCustomer(Customer customer)
{
using (SqlConnection con = new SqlConnection(CS))
{
var cmd = new SqlCommand("InsertCustomers", con);
con.Open();
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@FirstName", customer.FirstName);
cmd.Parameters.AddWithValue("@LastName", customer.LastName);
cmd.Parameters.AddWithValue("@Phone", customer.Phone);
cmd.Parameters.AddWithValue("@Email", customer.Email);
cmd.Parameters.AddWithValue("@Street", customer.Street);
cmd.Parameters.AddWithValue("@City", customer.City);
cmd.Parameters.AddWithValue("@State", customer.State);
cmd.Parameters.AddWithValue("@Zipcode", customer.Zipcode);
cmd.ExecuteNonQuery();
}
}
public void UpdateCustomer(Customer customer)
{
using (SqlConnection con = new SqlConnection(CS))
{
var cmd = new SqlCommand("UpdateCustomers", con);
con.Open();
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@CustomerId", customer.CustomerId);
cmd.Parameters.AddWithValue("@FirstName", customer.FirstName);
cmd.Parameters.AddWithValue("@LastName", customer.LastName);
cmd.Parameters.AddWithValue("@Phone", customer.Phone);
cmd.Parameters.AddWithValue("@Email", customer.Email);
cmd.Parameters.AddWithValue("@Street", customer.Street);
cmd.Parameters.AddWithValue("@City", customer.City);
cmd.Parameters.AddWithValue("@State", customer.State);
cmd.Parameters.AddWithValue("@Zipcode", customer.Zipcode);
cmd.ExecuteNonQuery();
}
}
}
}
Step 5: Open on Web Config file and add database connection string.
<connectionStrings>
<add name="connectionString" connectionString="data source=SHUBHAM\SQLEXPRESS; database=CrudMVC; integrated security=true;" providerName="System.Data.SqlClient"/>
</connectionStrings>
Step 6: Now right click on controllers folder and Add controller A window will appear. Choose MVC5 Controller with read/write actions and click "Add".
After clicking on "Add", another window will appear with DefaultController. Change the name to CustomerController and click "Add". The CustomerController will be added under the Controllers folder.
Complete code of controller Class
using MvcWithRepositry.Models;
using MvcWithRepositry.Repository;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Web;
using System.Web.Mvc;
namespace MvcWithRepositry.Controllers
{
public class EmployeeController : Controller
{
private readonly RespoCustomer respocustomer = new RespoCustomer();
// GET: Employee
public ActionResult Index()
{
var customers = respocustomer.GetCustomers();
return View(customers);
}
// GET: Employee/Details/5
public ActionResult Details(int? id)
{
var employee = respocustomer.GetCustomerById(id);
return View(employee);
}
// GET: Employee/Create
public ActionResult Create()
{
return View();
}
// POST: Employee/Create
[HttpPost]
public ActionResult Create(Customer customer)
{
try
{
if (ModelState.IsValid)
{
respocustomer.InsertCustomer(customer);
return RedirectToAction("Index");
}
return View("Create", customer);
}
catch (Exception ex)
{
return View();
}
}
// GET: Employee/Edit/5
public ActionResult Edit(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
var customer = respocustomer.GetCustomerById(id);
if (customer == null)
{
return HttpNotFound();
}
return View(customer);
}
// POST: Employee/Edit/5
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit(Customer customer)
{
try
{
respocustomer.UpdateCustomer(customer);
return RedirectToAction("Index", "Employee");
}
catch
{
return View();
}
}
// GET: Employee/Delete/5
public ActionResult Delete(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
var customers = respocustomer.GetCustomerById(id);
if (customers == null)
{
return HttpNotFound();
}
return View(customers);
}
// POST: Employee/Delete/5
[HttpPost, ActionName("Delete")]
public ActionResult Deletes(int id)
{
try
{
respocustomer.DeleteCustomer(id);
return RedirectToAction("Index", "Employee");
}
catch (Exception ex)
{
return View("Index");
}
}
}
}
Step 7: Right-click on the Index method in EmployeeController. The "Add View" window will appear with default index name checked (use a Layout page). Click on "Add.
Index View
@model List<MvcWithRepositry.Models.Customer>
@{
ViewBag.Title = "Index";
}
<script src="~/Scripts/bootstrap.js"></script>
<script src="~/Scripts/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<h3>Customer Details</h3>
<table id="example" class="table table-striped" style="margin-top: 25px;">
<thead class="mdb-color darken-3">
<tr class="text-white">
<th>@Html.DisplayNameFor(model => model[0].FirstName)</th>
<th>@Html.DisplayNameFor(model => model[0].LastName)</th>
<th>@Html.DisplayNameFor(model => model[0].Phone)</th>
<th>@Html.DisplayNameFor(model => model[0].Email)</th>
<th>@Html.DisplayNameFor(model => model[0].Street)</th>
<th>@Html.DisplayNameFor(model => model[0].City)</th>
<th>@Html.DisplayNameFor(model => model[0].State)</th>
<th>@Html.DisplayNameFor(model => model[0].Zipcode)</th>
<th>Action</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>@item.FirstName</td>
<td>@item.LastName</td>
<td>@item.Phone</td>
<td>@item.Email</td>
<td>@item.Street</td>
<td>@item.City</td>
<td>@item.State</td>
<td>@item.Zipcode</td>
<td>
<a href="@Url.Action("Details","Employee",new { id=item.CustomerId})" class="btn btn-sm btn-primary"><i class="fa fa-eye"></i></a>
<a href="@Url.Action("Edit","Employee",new { id=item.CustomerId})" class="btn btn-sm btn-info"><i class="fa fa-pencil-square"></i></a>
<a href="@Url.Action("Delete","Employee",new { id=item.CustomerId})" class="btn btn-sm btn-danger"><i class="fa fa-trash-o"></i></a>
</td>
</tr>
}
</tbody>
</table>
<div>
<a href="@Url.Action("Create","Employee")" class="btn btn-sm btn-primary">Create Customer</a>
</div>
Step 8: Now similarly right click on Details of ActionResult choose "Add View" and click on it. Now you will get another window which has default view name as ActionResult name. Checked Use a lay page and click on "Add".
Details View
@model MvcWithRepositry.Models.Customer
@{
ViewBag.Title = "Customers Details";
}
<div>
<h3>Customer Details</h3>
<hr />
<dl class="dl-horizontal">
<dt>
@Html.DisplayNameFor(model => model.FirstName)
</dt>
<dd>
@Html.DisplayFor(model => model.FirstName)
</dd>
<dt>
@Html.DisplayNameFor(model => model.LastName)
</dt>
<dd>
@Html.DisplayFor(model => model.LastName)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Phone)
</dt>
<dd>
@Html.DisplayFor(model => model.Phone)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Email)
</dt>
<dd>
@Html.DisplayFor(model => model.Email)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Street)
</dt>
<dd>
@Html.DisplayFor(model => model.Street)
</dd>
<dt>
@Html.DisplayNameFor(model => model.City)
</dt>
<dd>
@Html.DisplayFor(model => model.City)
</dd>
<dt>
@Html.DisplayNameFor(model => model.State)
</dt>
<dd>
@Html.DisplayFor(model => model.State)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Zipcode)
</dt>
<dd>
@Html.DisplayFor(model => model.Zipcode)
</dd>
</dl>
</div>
<p>
@Html.ActionLink("Edit", "Edit", new { id = Model.CustomerId }) |
@Html.ActionLink("Back to List", "Index")
</p>
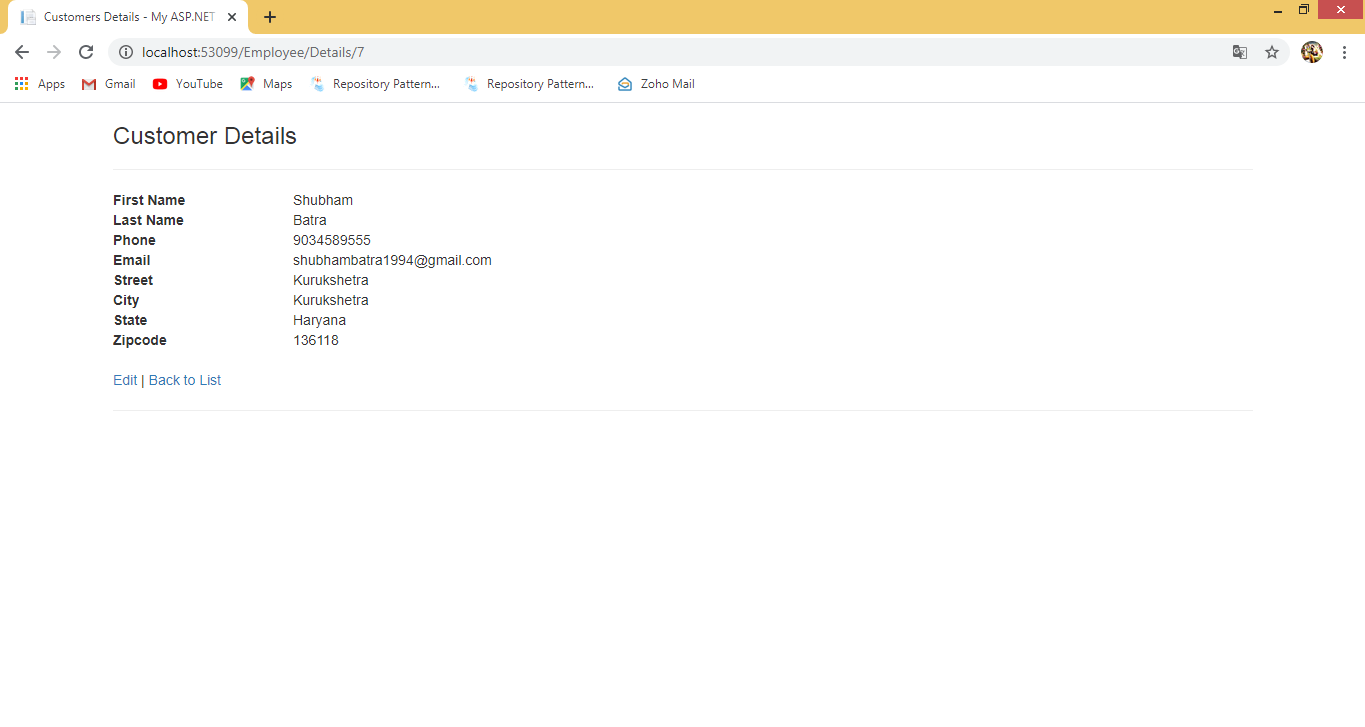
Step 9: Now click on Create of ActionResult choose "Add View" and click on it. Now you will get another window that has a default view name as ActionResult name. Checked Use a layout page and click on "Add".
Create View
@model MvcWithRepositry.Models.Customer
@{
ViewBag.Title = "Create";
}
<h3>Add Customer</h3>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.FirstName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.FirstName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.FirstName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.LastName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.LastName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.LastName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Phone, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Phone, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Phone, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Email, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Email, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Email, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Street, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Street, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Street, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.City, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.City, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.City, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.State, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.State, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.State, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Zipcode, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Zipcode, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Zipcode, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
}
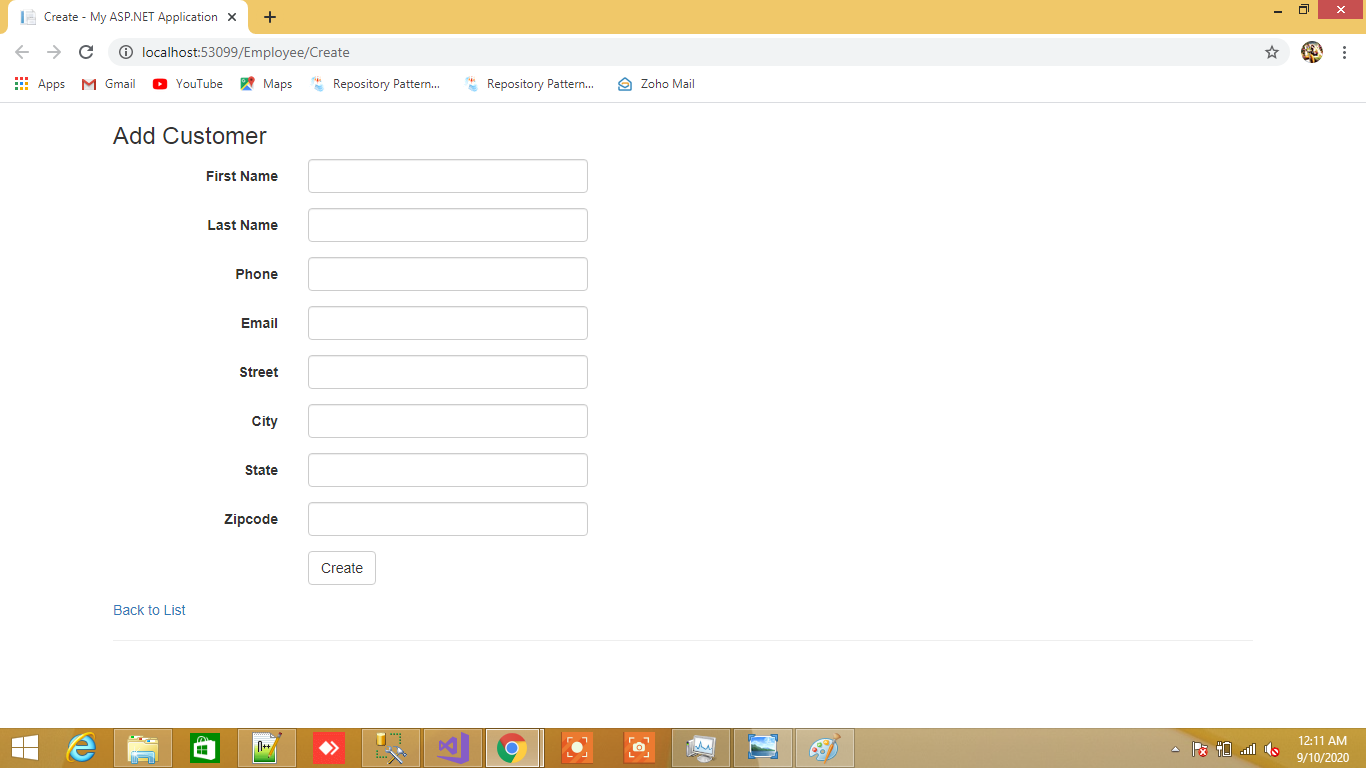
Step 10: Right click on Edit of ActionResult choose “Add View” and click on it. Now you will get another window which has default view name as ActionResult name. Checked Use a layout page and click on “Add”.
Edit View
@model MvcWithRepositry.Models.Customer
@{
ViewBag.Title = "Edit";
}
<h3>Customer Edit</h3>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
@Html.HiddenFor(model => model.CustomerId)
<div class="form-group">
@Html.LabelFor(model => model.FirstName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.FirstName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.FirstName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.LastName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.LastName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.LastName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Phone, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Phone, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Phone, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Email, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Email, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Email, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Street, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Street, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Street, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.City, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.City, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.City, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.State, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.State, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.State, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Zipcode, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Zipcode, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Zipcode, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Save" class="btn btn-default" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
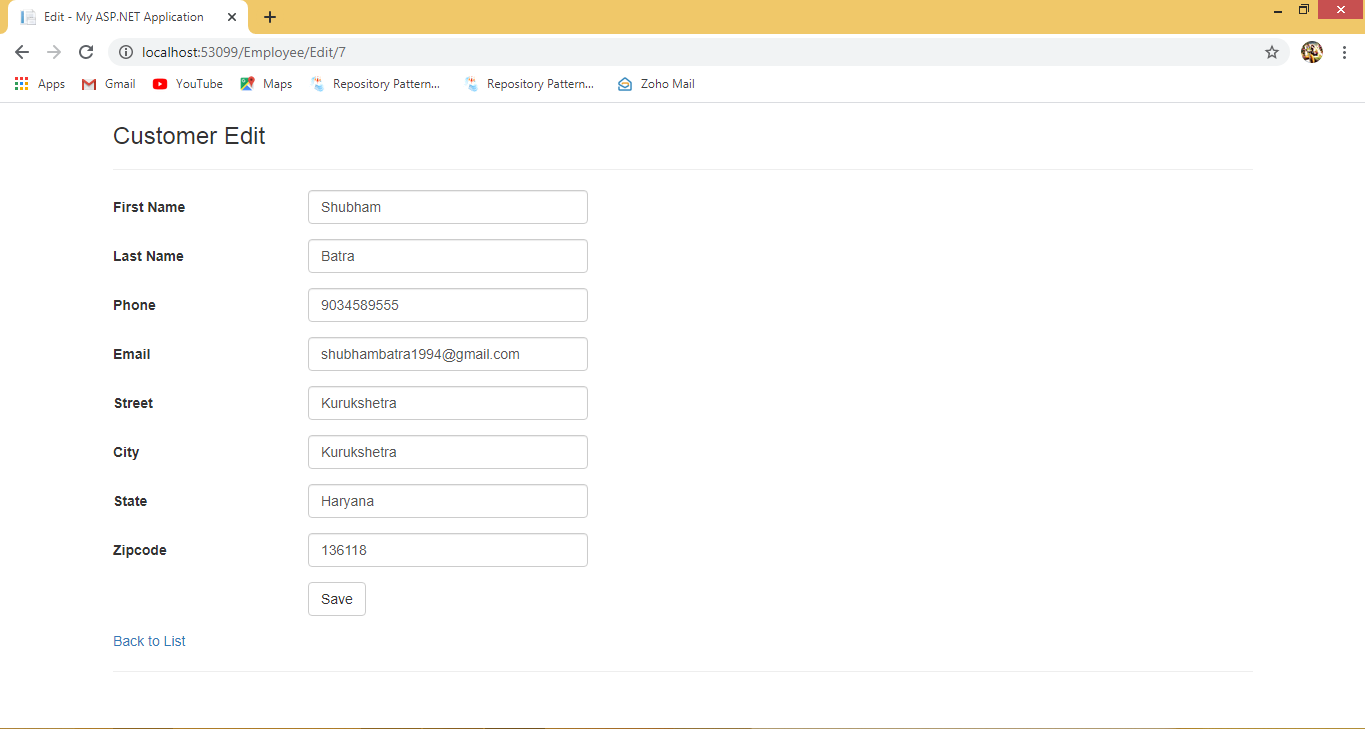
Step 11: Right click on Delete of ActionResult choose “Add View” and click on it. Now you will get another window which has default view name as ActionResult name. Checked Use a lay page and click on “Add”.
Delete View
@model MvcWithRepositry.Models.Customer
@{
ViewBag.Title = "Delete";
}
<h2>Customer Delete</h2>
<h4>Are you sure you want to delete this?</h4>
<div>
<hr />
<dl class="dl-horizontal">
<dt>
@Html.DisplayNameFor(model => model.FirstName)
</dt>
<dd>
@Html.DisplayFor(model => model.FirstName)
</dd>
<dt>
@Html.DisplayNameFor(model => model.LastName)
</dt>
<dd>
@Html.DisplayFor(model => model.LastName)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Phone)
</dt>
<dd>
@Html.DisplayFor(model => model.Phone)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Email)
</dt>
<dd>
@Html.DisplayFor(model => model.Email)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Street)
</dt>
<dd>
@Html.DisplayFor(model => model.Street)
</dd>
<dt>
@Html.DisplayNameFor(model => model.City)
</dt>
<dd>
@Html.DisplayFor(model => model.City)
</dd>
<dt>
@Html.DisplayNameFor(model => model.State)
</dt>
<dd>
@Html.DisplayFor(model => model.State)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Zipcode)
</dt>
<dd>
@Html.DisplayFor(model => model.Zipcode)
</dd>
</dl>
@using (Html.BeginForm("Delete", "Employee", FormMethod.Post, new { id = "DeleteCustomer", enctype = "multipart/form-data", name = "Delete" }))
{
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" id="Delete" formmethod="post" name="command" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
<div id="DeleteDialog">You really want to delete?</div>
@section scripts{
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$(document).ready(function () {
debugger;
$("#DeleteDialog").dialog({
height: 280,
modal: true,
autoOpen: false,
buttons: {
'Confirm': function () {
$("#DeleteCustomer").submit();
$(this).dialog('close');
},
'Cancel': function () {
$(this).dialog('close');
}
}
});
$("#DeleteDialog").hide();
$("#Delete").click(function (e) {
debugger;
$("#DeleteDialog").dialog("open");
e.preventDefault();
return false;
});
});
</script>
}
</div>
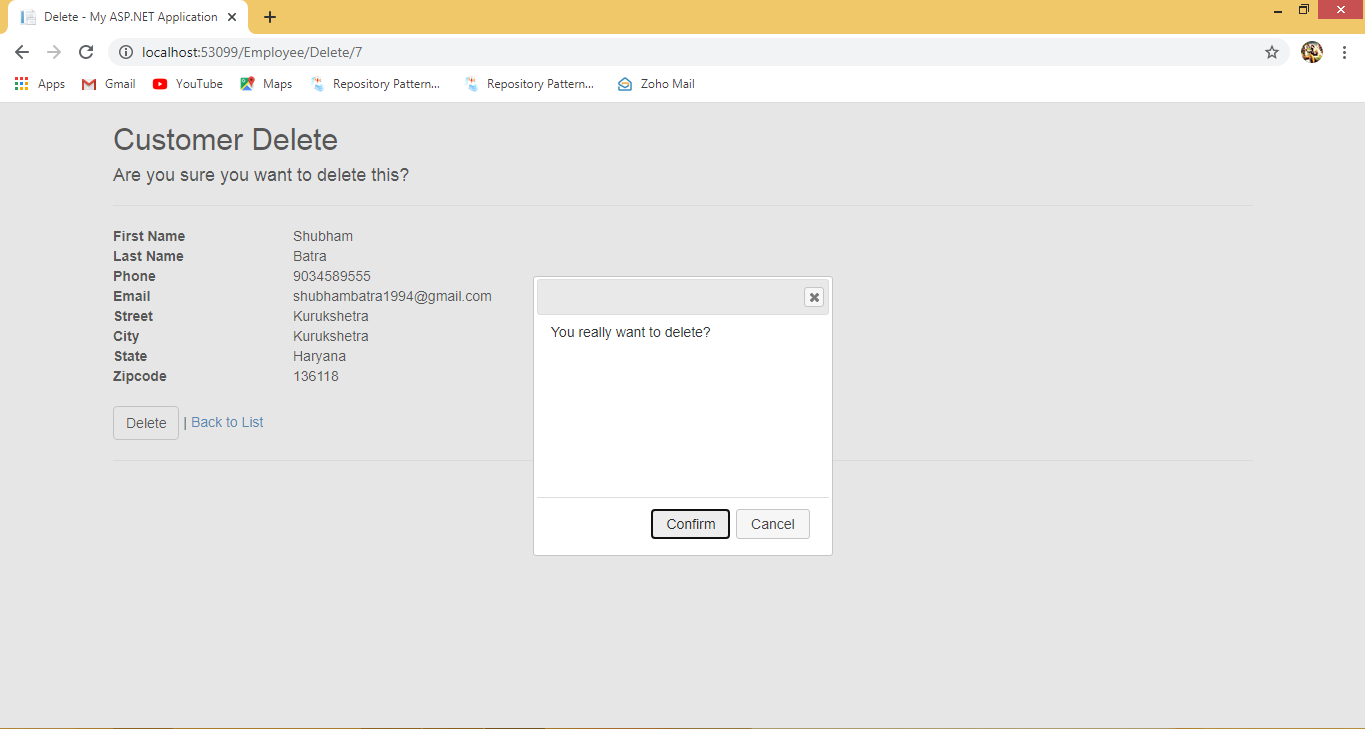