Authentication for swagger UI in production in ASP.Net Core 6.0
Add a new class called SwaggerBasicAuth and add the following code.
public class SwaggerBasicAuth { private readonly RequestDelegate next; public SwaggerBasicAuth(RequestDelegate next) { this.next = next; } public async Task InvokeAsync(HttpContext context) { if (context.Request.Path.StartsWithSegments("/swagger")) { string authHeader = context.Request.Headers["Authorization"]; if (authHeader != null && authHeader.StartsWith("Basic ")) { // Get the credentials from request header var header = AuthenticationHeaderValue.Parse(authHeader); var inBytes = Convert.FromBase64String(header.Parameter); var credentials = Encoding.UTF8.GetString(inBytes).Split(':'); var username = credentials[0]; var password = credentials[1]; // validate credentials if (username.Equals("Swagger") && password.Equals("Shubham123")) { await next.Invoke(context).ConfigureAwait(false); return; } } context.Response.Headers["WWW-Authenticate"] = "Basic"; context.Response.StatusCode = (int)HttpStatusCode.Unauthorized; } else { await next.Invoke(context).ConfigureAwait(false); } } } public static class SwaggerExtensions { public static IApplicationBuilder UseSwaggerAuthorized(this IApplicationBuilder builder) { // Your middleware registration goes here return builder.UseMiddleware<SwaggerBasicAuth>(); } }
Open the program.cs file and add the following code
builder.Services.AddSwaggerGen(c => { c.SwaggerDoc("v1", new Microsoft.OpenApi.Models.OpenApiInfo { Title = "SwaggerAuth", Version = "v1" }); });
app.UseSwaggerAuthorized(); app.UseSwagger(); app.UseSwaggerUI(c => { c.SwaggerEndpoint("/swagger/v1/swagger.json", "SwaggerAuth V1"); });
Complete code of the program.cs
using SwaggerAuth; var builder = WebApplication.CreateBuilder(args); // Add services to the container. builder.Services.AddControllers(); // Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle builder.Services.AddEndpointsApiExplorer(); builder.Services.AddSwaggerGen(c => { c.SwaggerDoc("v1", new Microsoft.OpenApi.Models.OpenApiInfo { Title = "SwaggerAuth", Version = "v1" }); }); var app = builder.Build(); // Configure the HTTP request pipeline. app.UseSwaggerAuthorized(); app.UseSwagger(); app.UseSwaggerUI(c => { c.SwaggerEndpoint("/swagger/v1/swagger.json", "SwaggerAuth V1"); }); app.UseHttpsRedirection(); app.UseAuthorization(); app.MapControllers(); app.Run();
Ensure that
UseSwaggerAuthorized()
is added before the calls to UseSwagger()
and UseSwaggerUI()
, so that the authentication middleware is invoked prior to accessing the Swagger UI.
Press F5 to run. You will notice that the Swagger page is loading, but the browser is prompting for credentials.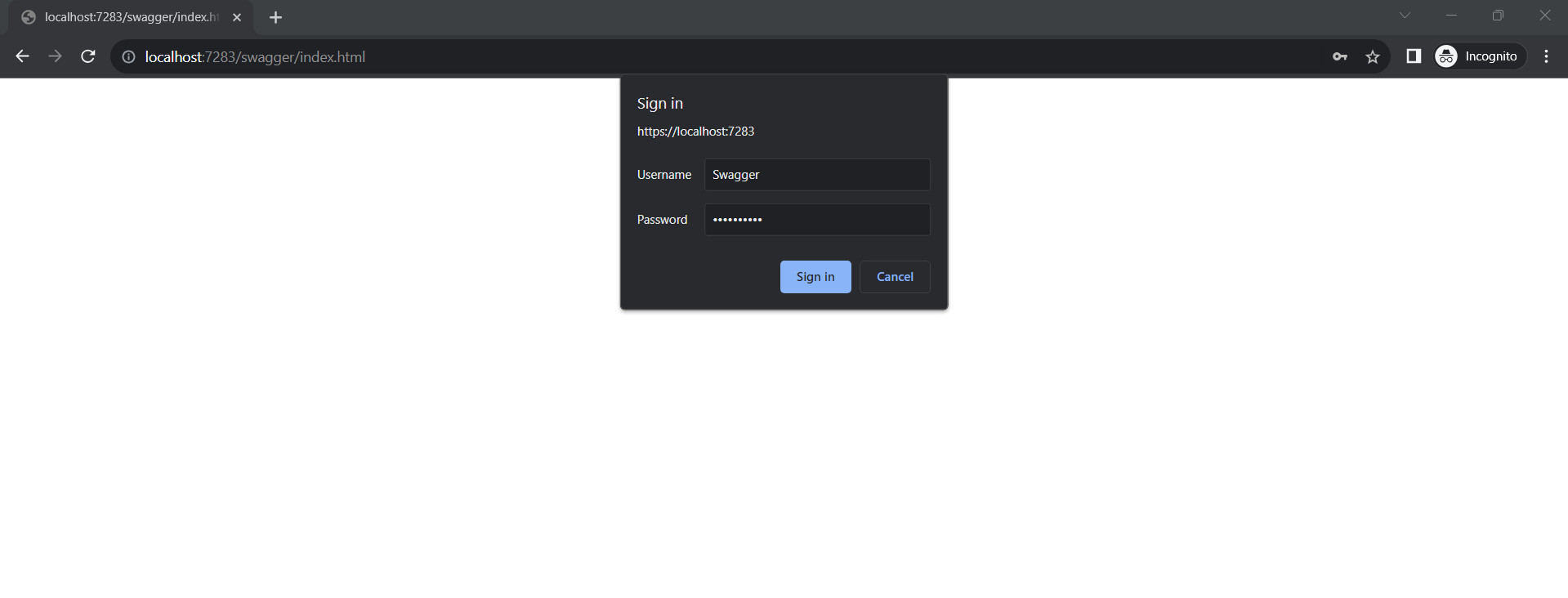
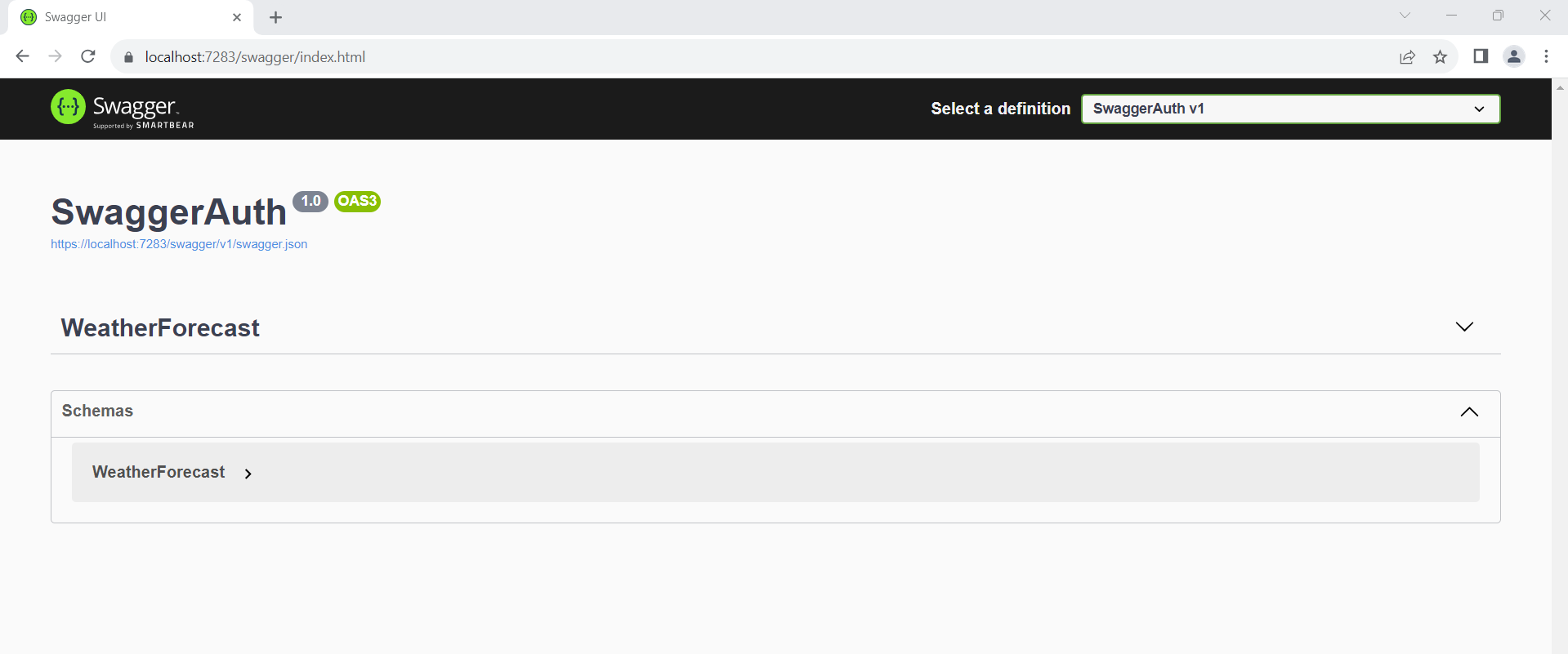